In this blog, I will explain the numbers in Python. What are the different types of number datatypes in Python? What are the functions we can perform in numbers in Python? So, let’s get started with the blog.
Table of Contents
- Introduction to Numbers in Python
- Different datatypes of Numbers in Python
- Number System in Python
- Type Conversion in Python
- Python Random Module
- Python Mathematics
- FAQ Related Questions
Introduction to Numbers in Python
Numbers in Python are used to store numeric values.
Numbers are immutable datatypes in Python, which means that changing the value of the number of data types results in newly allocated objects.
It supports integers, floating-point, and complex numbers. It can also be defined as an int, float, or complex.
- int – holds signed integers value of non-limited length.
- float – holds decimal value and it’s accurate up to 15 decimal places.
- complex – holds complex numbers
.
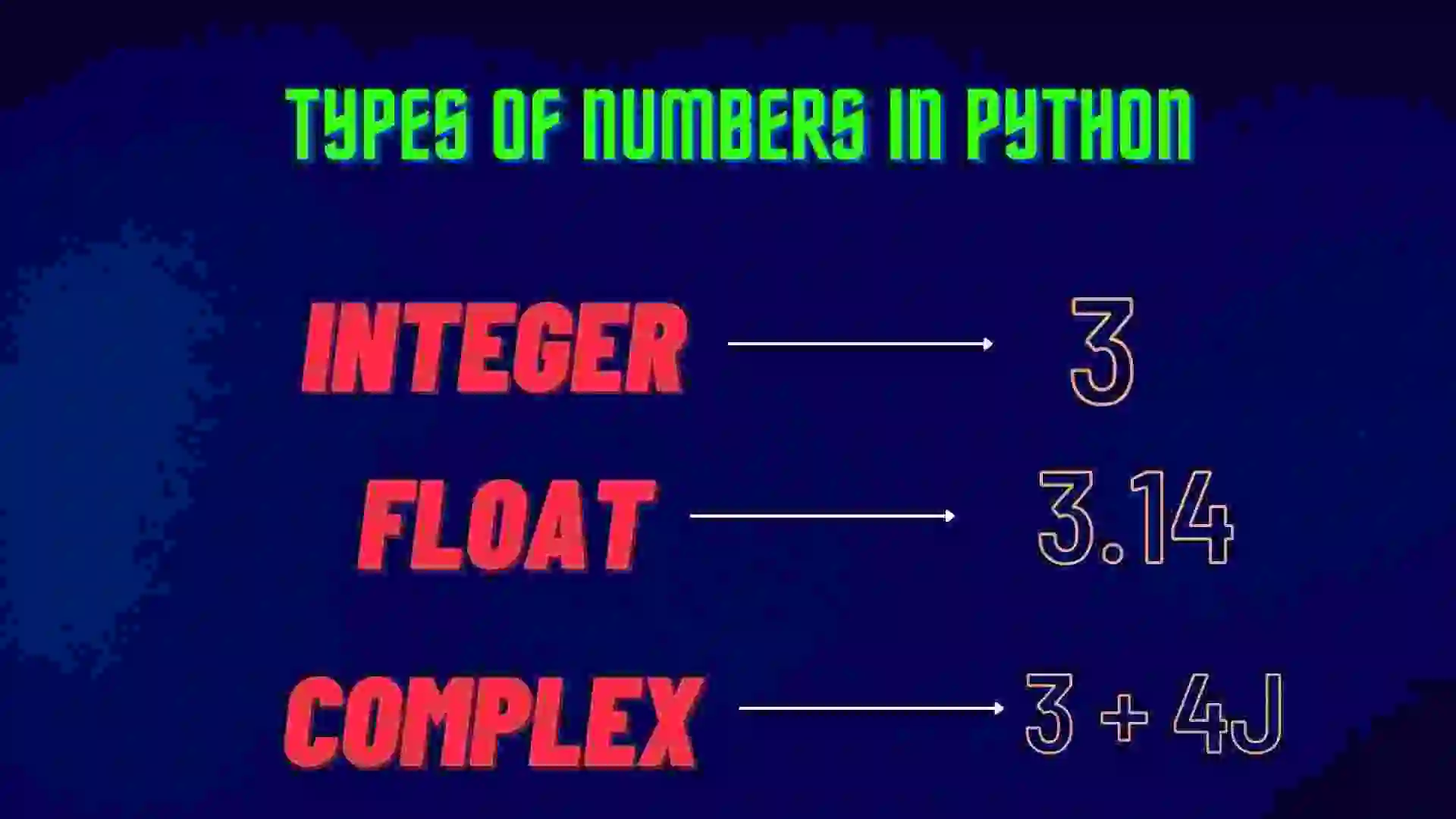
Different datatypes of Numbers in Python
A) Integer Datatype
Integer datatype includes the whole number, including negative numbers but not fractions. In Python, there is no limit to how long an integer value can be.
"""Integer"""
num1 = 8
num2 = -8
print(type(num1))
# Output:
<class 'int' >
print(type(num2))
# Output:
<class 'int' >
In the above example, we can clearly conclude that int datatypes can hold both positive and negative values.
B) Float Datatype
- A floating number has decimal point to indicate it.
- To designate scientific notation, the letters e or E may be added after a positive or negative number. 0.5 and -7.823457 are two examples of numbers that are represented as floats.
- A floating number can be positive or negative, but with a decimal point.
- They can be produced either manually by entering a number with a decimal point or by performing operations on integers, such as division.
- Any extra zeros at the end of the number are automatically ignored.
- A floating number in python has an accuracy of up to 15th decimal places, the 16th place can be accurate.
num1 = 8.5
num2 = -8.3
num3 = 5/4
num4 = 3 * 1.5
num5 = 2.5 * 2.5
print(type(num1))
print(type(num2))
print(type(num3))
print(type(num4))
print(type(num5))
## Output:
<class 'float'>
<class 'float'>
<class 'float'>
<class 'float'>
<class 'float'>
Here, num1 and num2 show that float numbers can be positive or negative.
From the above code, we can conclude that num3 shows that dividing any two integers produces a float.
From the above code, we can conclude that when running any operation on num5 (two float variables) or num4 (one float and one integer), it produces a float integer.
C) Complex Number Type
- Real and imaginary numbers are the two parts of the complex type number in Python.
- The imaginary number is nothing but the square root of -1. A complex number is not used more in programming.
- For imaginary numbers, only the letter j is valid.
For example:
The complex number 2+3j, here 2 is a real number and 3 multiplied by j is an imaginary number
num1 = 3+5j
num2 = 5j
print(type(num1))
print(type(num2))
## Output:
<class 'complex'>
<class 'complex'>
From the above code, we can conclude that in num1, 3 is the real, and 5 is the imaginary number having the complex number type.
From the above code, we can conclude that in num2, 0 is the real, and 5 is the imaginary number having the complex number type.
Number System in Python
- The decimal (base 10) number system is used for the numbers that we deal with on a daily basis.
- However, binary (base 2), hexadecimal (base 16), and octal (base 8) number systems must be used when writing computer programs.
- These numbers can be represented in Python by suitably prefixing the number.
Prefix for the number system:
Number System | Prefix |
Binary Number | 0b or 0B (zero with b or B) |
Octal | 0o or 0O (zero with o or O) |
Hexadecimal | 0x or 0X (zero with x or X) |
Below are some examples of number systems:
print(0b1010101)
print(0o176)
print(0x23FA2)
## Output:
85
126
147362
From the above code, we can conclude that:
- For binary numbers, our number system starts with 0b, and the next number should only include 1 or 0. Other than that it will return an Error.
- For octal numbers, our number system starts with 0o, and the next number should include the number from 0 to 7. Other than that it will return an Error.
For hexadecimal numbers, our number system starts with 0x and the next number should include 0 to 7 or an alphabet from A to F. Other than that it will return an Error.
Type Conversion in Python
Type conversion is the process of converting from one type of number into another type. Type conversion is also known as “type casting” in programming.
There are two ways to convert one type of number to another type of number in Python.
A) Using Arithmetic Operations
Arithmetic Operations are the operation in which there are two or more operands are present and we have to perform an operation on that operand by using arithmetic operators like addition, subtraction, multiplication, etc.
When we use arithmetic operators the type of number implicitly or automatically changes.
num1 = 5
num2 = 2.5
num3 = 5*2.5
print(num3)
## Output:
12.5
From the above code, we can easily conclude that the num1 datatype is an int number datatype, while num2 is a float number datatype. When we store the output of num1 and num2, implicitly or automatically, it stores the num3 as a float number datatype.
Note: This method does not work on complex number datatypes in Python.
B) Using Built-in Python Functions
Python performs type casting internally during the execution of expressions and binds the values to their actual types; however, in rare situations, we may need to explicitly define the data type of a variable.
The following are the functions that are used to perform type conversion:
- int(number): It is used to convert a number into an integer number datatype.
- float(number): It is used to convert a number into a float number datatype.
- complex(number): It is used to convert a number into a complex number datatype, taking the number as a real number, and the default imaginary number will be zero.
- complex(real, imaginary): It is used to convert a number into a complex number datatype with a real and imaginary number.
# Convert to integer datatype
num1 = 3.6
print(int(num1))
## Output
3
From the above code, we can see that num1 is a float number datatype, but we have converted it to an integer number datatype by using the int() method. We cannot convert complex number datatypes to integer number datatypes.
# Convert to float datatype
num1 = 3
print(float(num1))
## Output:
3.0
From the above code, we can see that num1 is an integer number datatype, but we have converted it to a float number datatype by using the float() method. We cannot convert complex number datatypes to float number datatypes.
# Convert to Complex Datatype
num1 = 3
print(complex(num1))
print(complex(2, num1))
## Output:
(3+0j)
(2+3j)
num2 = 3.5
print(complex(num2))
print(complex(num2, 5))
## Output:
(3.5+0j)
(3.5+5j)
From the above code, we can clearly conclude that num1 is an integer datatype, while a complex function has two parameters: the first is for a real number, and the second is for an imaginary number. If we don’t pass the number in the second parameter, then it will take the value 0. That’s why complex(num1) gives an output of 3+0j and complex(2,num1) gives an output of 2+3j.
From the above code, we can clearly conclude that num2 is a float datatype, while a complex function has two parameters first is for real and the second number is for an imaginary number. If we don’t pass the number in the second parameter then it will take as 0. That’s why complex(num2) gives an output of 3.5+0j and complex(num2,5) gives an output of 3.5+5j.
Python Random Module
Python provides built-in random modules to generate random numbers or to pick a random item from an iterator.
For using the random module function first we have to import a random module into the program.
A) choice()
It returns the random item from the passed list, tuple, and dictionary in Python.
Syntax:
choice(seq)
import random
emplist = ["Alex", "Copper", "Sam", "Travis"]
print(random.choice(emplist))
## Output
Sam
B) randrange()
It returns the random element from the specific range.
Syntax:
randrange(start, stop, step)
import random
print(random.randrange(12, 24, 3))
## Output:
18
C) random()
It returns the random value which is greater than 0 and less than 1.
import random
# emplist = ["Alex", "Copper", "Sam", "Travis"]
# print(random.choice(emplist))
print(random.random())
## Output:
0.3286
D) shuffle()
It shuffles the list of objects passed to it.
import random
emplist = ["Alex", "Copper", "Sam", "Travis"]
random.shuffle(emplist)
print(emplist)
## Output:
["Copper", "Sam", "Travis","Alex"]
E) uniform()
A random integer that is larger than or equal to x and less than y is generated.
Syntax:
uniform(x,y)
import random
print(random.uniform(30, 50))
## Output:
34.7886
Conclusion of all the functions in the table
Functions | Description |
choice(seq) | It returns the random item from the passed list, tuple, and dictionary in Python. |
randrange(start,stop,step) | It returns a random element from a given range. |
random() | It returns the random value that is greater than 0 and less than 1. |
shuffle(list) | It shuffles the list of objects passed to it. |
uniform(x,y) | A random integer that is larger than or equal to x and less than y is generated. |
Python Mathematics
Python provides built-in math modules so we can perform many difficult mathematical operations like statistics, trigonometry, probability, logarithms, etc.
For using the math module function, first, we have to import a math module into the program.
A) abs()
It returns the positive distance between 0 and x. If you pass a negative value, it returns its positive value of it.
print(abs(-3))
## Output
3
B) ceil()
The ceiling value of x is the smallest integer that is not less than x.
import math
print(math.ceil(3.5))
print(math.ceil(3.1))
## Output
4
4
C) exp()
The exponent value of x that is ex.
import math
print(math.exp(2))
## Output
7.38905609893065
D) floor()
The floor value of x, i.e., the largest integer that is less than x
import math
print(math.floor(3.5))
print(math.floor(3.1))
## Output
3
E) log()
The natural log value of x.
import math
print(math.log(3))
## Output
1.0986122886681098
F) max()
It returns the maximum number from the passed sequence list.
print(max([3, 5, 2, 1]))
## Output
5
G) min()
It returns the minimum number from the passed sequence list.
print(min([3, 5, 2, 10]))
## Output
2
H) modf()
The result is a tuple that includes a floating point number’s fractional and integer components. Also returned as a float is the integer component.
import math
print(math.modf(7))
## Output
(0.0, 7.0)
I) pow()
It returns the x ** y.
import math
print(math.pow(2, 3))
## Output
8.0
J) round()
The value of x is rounded to n digits.
print(round(2.879797, 3))
## Output
2.88
K) sqrt()
It returns the square root of value x.
import math
print(math.sqrt(32))
## Output
5.656854249492381
Conclusion of all the functions in the table.
Function | Description |
abs(x) | Returns the positive distance between 0 and 1. If you pass a negative value it returns its positive value of it. |
ceil(x) | The ceiling value of x, i.e., the smallest integer that is not less than x. |
exp(x) | The exponent value of x that is ex. |
floor(x) | The floor value of x, i.e., the largest integer that is less than x |
log(x) | The natural log value of x. |
max(x1,x2,…) | It returns the maximum number from the passed sequence list. |
min(x1,x2,…) | It returns the minimum number from the passed sequence list. |
modf(x1,x2,….) | The result is a tuple that includes a floating point number’s fractional and integer components. Also returned as a float is the integer component. |
pow(x,y) | t returns the x ** y. |
round(x.[n]) | The value of x is rounded to n digits. |
sqrt(x) | It returns the square root of value x. |
FAQ Related Questions
Numbers in Python support integer, floating-point, and complex numbers. It can also be defined as int, float, and complex.
1) int – holds signed integers value of non-limited length.
2) float – holds decimal value and it’s accurate up to 15 decimal places.
3) complex – holds complex numbers.
1) Numbers in Python are used to store numeric values.
2) Numbers in Python are immutable datatypes, which means that changing the value of the number of data types results in newly allocated objects.
Python provides built-in random modules to generate random numbers or to pick a random item from an iterator.
To use the random module function first we have to import a random module into the program.
1) By using arithmetic operations on numbers in Python
2) By using the built-in python function.
Floor: The floor value of x, i.e., the largest integer that is less than x
Ceil: The ceiling value of x, i.e., the smallest integer that is not less than x.