In your mind there are many several questions will be there about loops in python programming. What is a loop in python and why the programmers used loops in their programs? What is the advantage of using a loop? What are the different types of loops in python? So, let’s get started with your answers.
Table of Contents
- Loops in Python
- Application of Loops in Python
- Types of Loops in Python:
- Range() in Python
- Difference between for-loop and while-loop in Python
- FAQ related to Loop in Python
Loops in Python
A loop in Python is a sequence of instructions that is repeated until it satisfies a certain condition that breaks the iteration. Loop in Python reduces the number of lines of code in our program.
Application of Loops in Python
- Reduces the number of lines of code.
- Due to fewer lines of code, make the programmer easy to understand.
- Time-consuming.
For example, Your teacher had told you to write a “Good morning …” sentence 100 times, so you have to write 100 times that sentence. This process consumes more time and takes 100 lines of code to complete these tasks. Now, these processes can be solved within 3 lines of code and it can save you a lot of valuable time. That’s why we use the loop in the program.
Types of Loops in Python:
So, basically, there are two types of loops in python:
- for loop
- while loop
- Nested Loop
For Loop
for loop in python is used for iterating over a sequence that is either in List, Tuples, Dictionary, Set, or a String.
With the help of for loop, we can execute a set of statements, once for each item in the dictionary, list, etc.
Syntax:
for iterate_var in sequence:
statement
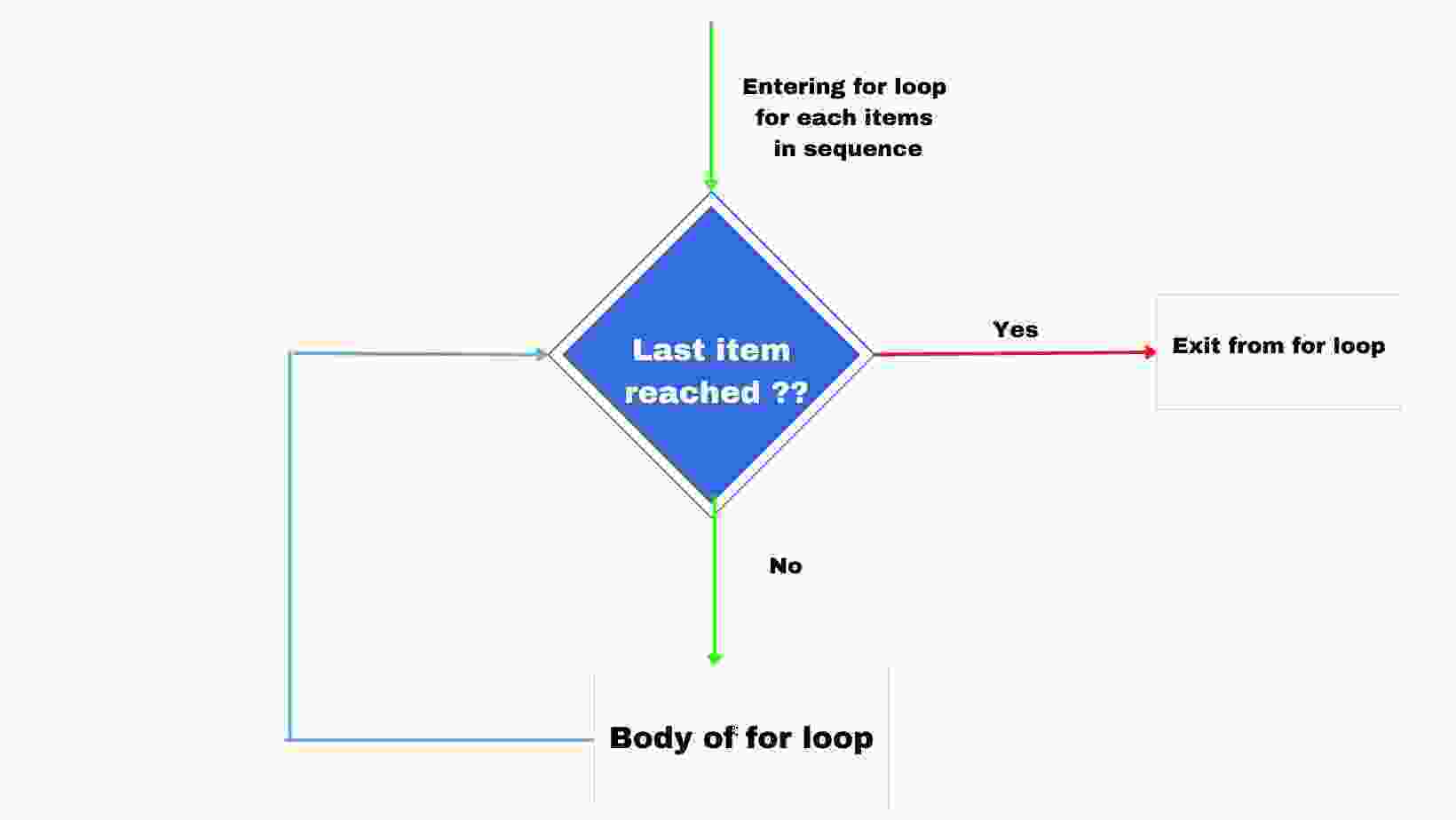
"""For loop"""
""""In List"""
list1 = ["Sam", "Tom", "Jerry", "Oscar"]
for i in list1:
print(i)
#Output :
Sam
Tom
Jerry
Oscar
So, here we can clearly see how the for loop is iterating each element in an array. Here “i” is playing the role of the index’s value. That means at the 0th index the value of the list1 array is “Sam”, at the 1st index the value of the list1 array is “Tom” and so on.
Let’s check what will happen if I use the loop in a list containing a list of elements.
"""For loop"""
""""List of list"""
list2 = [["Tom", 62], ["Sam", 92], ["Jerry", 90], ["Oscar", 98]]
for player, run in list2:
print("Player", player, "has made run", run)
#Output :
Player Tom has made run 62
Player Sam has made run 92
Player Jerry has made run 90
Player Oscar has made run 98
So here we can see the list contains a list of elements, and for iteration, we are using two variables player and run in the list2. Here, the player iterates through each item of every first element of an array, For example in the first list, it carries the result of “Tom” and the next iteration “Sam” and so on. While run carries the second element of each list. On the first iteration, it carries the result of 62, and on the next iteration 92, and so on.
For loop in python Dictionary
"""For loop"""
""" Dictionary"""
dict1 = {'tom': 63, 'jerry': 89, 'oscar': 98, 'patrick': 76}
for student in dict1:
print(student) # It will print only keys not values
#Output:
tom
jerry
oscar
patrick
# for printing values
for student, marks in dict1.items():
print(student, "has got marks", marks)
#Output :
tom has got marks 63
jerry has got marks 89
oscar has got marks 98
patrick has got marks 76
Now, we check how we use for loop in the python dictionary, so first of all we should know that the dictionary store the result in key-value pair. Let’s start, Here the student is the iterator in for loop is take only the result of the key and does not include the result of the value. For example, while iterating the for loop in the python dictionary we got the result tom in the first iterator and jerry in the second iterator, and so on.
So, now how do we store the result of value in the dictionary? So we have to use the “items()” function of the dictionary. Now “student” and “marks” get the result of key and value respectively.
For loop in python Tuples
"""For loop"""
"""Tuples"""
tuples1 = ("Tom", "Jerry", "Oscar", "Alex")
for t in tuples1:
print(t)
#Output :
Tom
Jerry
Oscar
Alex
"""Tuples with dictionary"""
tuples2 = ({'tom': 63, 'jerry': 89, 'oscar': 98, 'patrick': 76})
for stu, marks in tuples2.items():
print("Studnet", stu, "have got", marks)
#Output :
Studnet tom have got 63
Studnet jerry have got 89
Studnet oscar have got 98
Studnet patrick have got 76
Now, we check how we use for loop in the python tuples, so first of all we should know that the tuples store the result in sequence and key-value pair. Let’s start, Here the t is the iterator in for loop is take only the result of the sequence’s value. For example, while iterating the for loop in the python tuples we got the result tom in the first iterator and jerry in the second iterator, and so on.
So we have to use the “items()” function of the tuples. Now “student” and “marks” get the result of key and value respectively.
For loop in python String
"""For loop"""
"""String"""
str1 = "Bin-FinTech"
for i in str1:
print(i, end=" ")
# B i n - F i n T e c h
Now, we have to use it in a for-loop in Python’s String. So, the string is a collection of characters, so every iteration loop will take single elements from the string. So, in the first iteration, it takes the value of “B” and in the second iteration, “i”, and so on.
While-loop in Python
While loop in Python requires a variable to start the iteration.
With the while loop in python, we can execute a set of statements as long as a condition is true.

Syntax:
while expression:
statement
"""While loop"""
list1 = ["Sam", "Tom", "Jerry", "Oscar"]
i = 0
while (i < len(list1)):
print(list1[i], end=" ")
i = i+1 ## Incrementing the value of i
#Output:
Sam Tom Jerry Oscar
So here, to start the loop we have created one variable which will be used to check whether the condition is satisfied or not. “len()” returns the length of the list. So, in the first iteration “i” have the value of 0, so it will check the condition i.e, 0 < 4 (the length of the list1), it satisfies the condition it will go under the while loop print the result and now it will increment the value of “i” it will become 1. It will iterate till it does not satisfy the condition.
Nested Loop in Python
If loops exist inside the body of another loop, it is called a “nested loop.”
# Nested Loops
employee = ["Alex", "Tom", "Sam"]
car = ["BMW", "Ford", "Volvo"]
for x in employee:
for y in car:
print(x, "will ride a car", y)
#Output:
Alex will ride a car BMW
Alex will ride a car Ford
Alex will ride a car Volvo
Tom will ride a car BMW
Tom will ride a car Ford
Tom will ride a car Volvo
Sam will ride a car BMW
Sam will ride a car Ford
Sam will ride a car Volvo
Working of Nested Loops:
The program first encounters with the outer loop i.e employee and the first iterate is x and performs its first iteration. The first iteration triggers the inner loop i.e car having first iterate y. It will perform till it iterates the whole elements present in the list. That’s why we get the output:
Alex will ride a car BMW
Alex will ride a car Ford
Alex will ride a car Volvo
where Alex is the first value of the outer loop and BMW, Ford, and Volvo are the elements of the inner loop.
After completing the iteration of the inner loop, the program again returns to the outer loop and picks the second element of the outer loop, i.e., Tom, and again triggers the inner loop.
Tom will ride a car BMW
Tom will ride a car Ford
Tom will ride a car Volvo
The loop will iterate till iterates all the elements of the outer loop.
Range() in Python
The range() the function returns a sequence of numbers, starting from 0 by default, increments by 1 (by default), and stops before a specified number.
Syntax:
range(start,stop,step)
"""Range function loop"""
for i in range(0, 41, 5):
print(i, end=" ")
Output :
0 5 10 15 20 25 30 35 40
So, here loop starts from 0 and ends at 40 it does not include 41 in the loop and has an increment of 5 in the loop.
Difference between for-loop and while-loop in Python
For-loop in Python | While-loop in Python |
It is used for definite loops when the iteration of the loop is known. | It is used when the iteration of the loop is not known. |
It can have its counter variables declared in the declaration itself. | There is no built-in loop control variable |
It is preferable when we know how many times the loop will iterate. | It is preferable when we don’t have any idea of how much the time loop will iterate. |
The loop will iterate an infinite number of times if the condition is not specified. | If the condition is not specified then it will return a compilation error. |
Syntax: for iterate_var in sequence: statement | Syntax: while expression: statement |
FAQ related to Loop in Python
A loop in Python is a sequence of instructions that is repeated until it satisfies a certain condition that breaks the iteration. Looping in Python reduces the number of lines of code in our program.
1) for-loop
2) while-loop
3) nested loop
for iterate_var in sequence:
statement
while expression:
statement
For-loop: It is used for definite loops when the iteration of the loop is known.
While-loop: It is used when the iteration of the loop is not known.
1) Reduces the number of lines of code.
2) Due to fewer lines of code, makes the programmer easy to understand.
3) Time-consuming.