In this blog, I will discuss the Python dictionary. So don’t worry, I will not waste your time. I will explain what a dictionary is and why we use one in our program. Is the dictionary mutable or immutable? What operation is performed on the dictionary, and what valuable tips can you use in your programs? So let’s get started.
Table of Contents
- Introduction to Dictionary in Python
- Advantages of using a Dictionary
- Dictionary in Python is mutable or immutable
- Operations performed on Dictionary
- Python Dictionary Methods
- Difference between a List and a Dictionary
- FAQ
Introduction to Dictionary in Python
A Dictionary in Python is an ordered collection (Python 3.7), before that dictionary in python was an unordered collection. So, the dictionary is an ordered collection of items. It stores the values in the key-value format, where the key is a unique identifier that is associated with each value.
Unlike other datatypes, a dictionary in Python can also hold a single value.
Due to the key-value pair, it is more optimized than the other datatypes.
A dictionary is used when we have a huge amount of data.
# Dictionary in python
comapny_name = {"Bin-Fin", "Tech"}
print(comapny_name)
# Output:
{'Bin-Fin', 'Tech'}
emp_detail = {1: "Alex", 2: "Sam", 3: "Copper", 4: "Tom"}
print(emp_detail)
# Output:
{1: 'Alex', 2: 'Sam', 3: 'Copper', 4: 'Tom'}
Advantages of using a Dictionary
- By using Dictionary in Python, we can develop effective data structures.
- Records of combined data can be found in Dictionary in Python.
- Dictionary representations of sparse data structures are useful.
- For Dictionary in Python, since these operations have already been done, there is no need to manually write data search algorithms.
Dictionary in Python is mutable or immutable
Let me clarify that in Python, the dictionary is mutable. As we know, Dictionary stores the values in key-value format. So, let us understand what the key is and its purpose.
What are the keys in the dictionary?
- Keys are immutable i.e., they cannot be changed.
- The datatypes of keys in the dictionary are either numbers or strings.
- The Keys in the dictionary are unique and cannot be duplicated inside the dictionary.
- If it is used more than once then it will override the previous key’s value.
- The Key connects with the value in the dictionary.
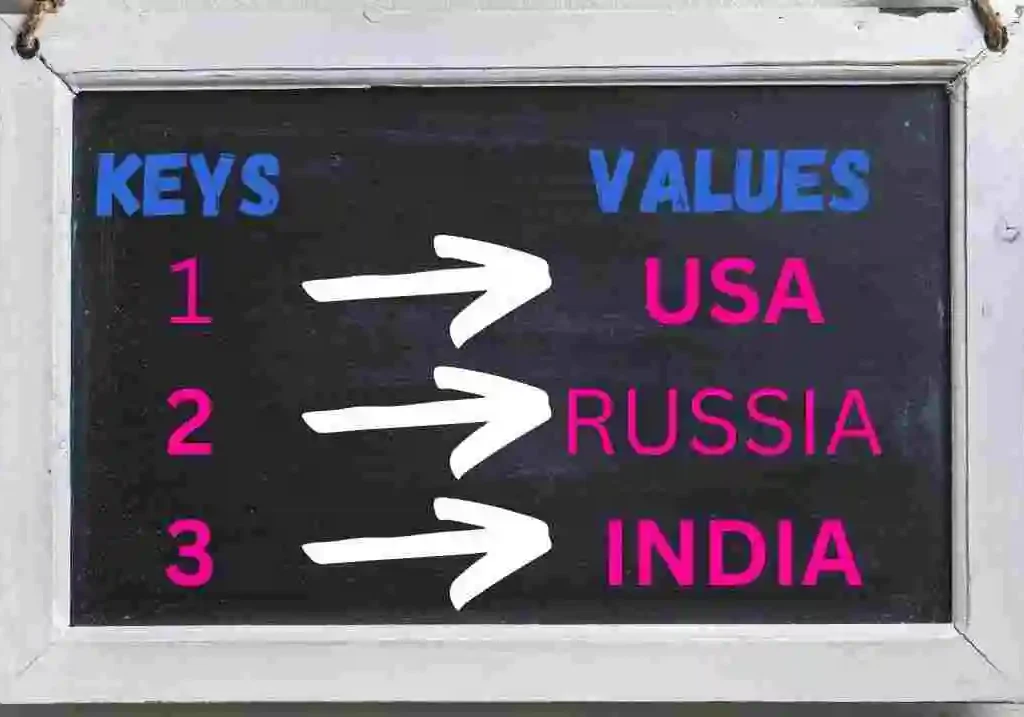
Operations performed on Dictionary
A) Creating a Dictionary in Python
- A dictionary can be created by using a sequence of elements inside the sequence of elements within the curly braces ({}) separated by a comma (,).
- It holds the pair value, one being is Key and the other one is value. {Key: value}
- Values can be of any datatype and can be duplicated, while keys are unique and immutable in the dictionary in Python.
- Keys are case-sensitive, the same name but different cases of the key will be treated differently. They will be considered two keys. For Example, bin and Bin are two different Keys.
# Creating a dictionary in python
1) comapny_name = {"Bin-Fin", "Tech"}
print(comapny_name)
# Output:
{'Bin-Fin', 'Tech'}
2) emp_detail = {1: "Alex", 2: "Sam", 3: "Copper", 4: "Tom"}
print(emp_detail)
# Output:
{1: 'Alex', 2: 'Sam', 3: 'Copper', 4: 'Tom'}
In example (1), we can clearly see that in the program I have not provided the key, only values. So, by default, the compiler will provide a key starting from 0. It will be considered as:
{‘0′:’Bin-Fin’, ‘1’:’Tech’}
In example (2), I have provided the key, where 1 is the key and it connects with the value “Alex”, key 2 connects with the value “Sam,” and so on.
Creating a nested dictionary in Python:
# Creating a nested dictionary
emp_detail = {1: 'Alex', 2: 'Sam', 3: {
'name': 'Tom', 'age': 45, 'location': 'USA'}}
print(emp_detail)
## Output:
{1: 'Alex', 2: 'Sam', 3: {'name': 'Tom', 'age': 45, 'location': 'USA'}}
Creating a dictionary using the dict() function
A dictionary in Python can be created by using the dict() function.
An empty dictionary can be created by using empty curly braces ({}).
# Creating a dictionary by using dict() function
1) emp_detail = {} # Empty dictionary
print(emp_detail)
# Output:
{}
2) emp_detail = dict([(1, "Alex"), (2, "Sam"), (3, "Tom")])
print(emp_detail)
#Output:
{1: 'Alex', 2: 'Sam', 3: 'Tom'}
In Example (1), we have created an empty dictionary by just using empty curly braces.
In Example (2), we have created a list and stored a sequence of elements, but, in the same line, we have converted the list to a dictionary by using the dict() function.
B) Adding an Element in the Dictionary
We can easily add elements to the dictionary with the help of square brackets by providing key names and values.
If the key is already present and you are again adding that same key, then it will override the value of that key.
Syntax: Dictionary_name[“Key_name”]=Value
# Adding Element in dictionary
1) country_detail = {}
print("Initial Dictionary : ", country_detail)
## Output:
Initial Dictionary : {}
2) country_detail["USA"] = "Washington D.C"
country_detail["Russia"] = "Moscow"
print("Final Dictionary : ", country_detail)
## Output:
Final Dictionary : {'USA': 'Washington D.C', 'Russia': 'Moscow'}
In example (1), we have created an empty dictionary.
Now we are adding the two keys, i.e USA and Russia so, we have provided the key and values respectively.
country_detail[“USA”] = “Washington D.C”
country_detail[“Russia”] = “Moscow”
Now you can see the final output, i.e., we have added the two keys successfully.
We can also add or update the element in the dictionary by using update().
The syntax for the update function in the dictionary is:
Dictionary_var.update({key:value})
# Adding Element in dictionary
1) country_detail = {}
country_detail["USA"] = "Washington D.C"
country_detail["Russia"] = "Moscow"
print("Initial Dictionary : ", country_detail)
# Output:
Initial Dictionary : {'USA': 'Washington D.C', 'Russia': 'Moscow'}
2) country_detail.update({"USA": "DC"})
country_detail.update({"India": "Delhi"})
print("Update Dictionary : ", country_detail)
#Output:
Update Dictionary : {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
In example (1), we created an empty dictionary and added the two keys, i.e., USA and Russia.
Now for the update in the dictionary, we have used the update function in example (2). Firstly, in the previous directory, there was a key for “USA.” In the update, we are again using the USA key, so this time it will override that key and value.
Secondly, we are adding a new key India. That means it is doing two things:
- If the key is present in the dictionary then it will override that key which means it is updating the key value.
- If the key is not present then it will add that key to the dictionary.
C) Accessing an Element of Dictionary:
We can easily access the elements of a dictionary providing a key in the square brackets.
Syntax:
dictionary_var[“Key_name”]
# accessing the elements
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
print(country_detail['USA'])
#Output:
DC
print(country_detail["UK"])
#Output:
Traceback (most recent call last):
File "dictionary.py", line 61, in <module>
print(country_detail['UK'])
KeyError: 'UK'
If the key is not present in the dictionary, and you are accessing that key, it will return a Key Error.
Another way to access the elements of the dictionary is by using the get() function.
Syntax:
Dictionary_var.get(“Key_name”)
# accessing the elements
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
print(country_detail.get("USA"))
#Output:
DC
print(country_detail.get("US"))
#Output:
None
If the key is not present in the dictionary, then it will not return an Error, It will return the value None.
Accessing an Element in a Nested Dictionary:
In a Nested Dictionary, we can easily access the elements of a dictionary by using the index [] operator.
# Nested Python dictionary
employee_detail = {
'1': {'Name': "Alex", "Age": 23},
'2': {'Name': "Tom", "Age": 24},
'3': {'Name': "Sam", "Age": 42}
}
print(employee_detail['1']["Name"])
#Output:
Alex
print(employee_detail['2']["Age"])
#Output:
24
print(employee_detail['3']["Name"])
#Output:
Sam
D) Deleting an Element of the Dictionary
We can delete the element of a dictionary by using the del keyword and square brackets with the key value.
Syntax:
del Dictionary_car[“key”]
# Deleting an element in dictionary
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
1) del country_detail['Russia']
print(country_detail)
#Output:
{'USA': 'DC', 'India': 'Delhi'}
2)del country_detail['UK']
#Output
Traceback (most recent call last):
File "dictionary.py", line 71, in <module>
del country_detail['UK']
KeyError: 'UK'
In example 1, key Russia is deleted from the dictionary by using the del keyword. In example 2, if the key is not present in the dictionary, then it will return a Key Error.
E) Membership Operator in Dictionary
With the help of the membership operator, we can check whether the key is present or not in the dictionary.
It is only used for the keys, not for the values.
If the key is present in the dictionary, it will return true otherwise false.
# Membership operator in dictionary
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
print('USA' in country_detail)
#Output
True
print('UK' in country_detail)
#Output
False
Python Dictionary Methods
A) clear() :
Remove all the elements from the dictionary in Python.
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
print(country_detail.clear())
#Output
None
B) copy():
It returns a new copy of the dictionary.
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
copy_country_detail = country_detail.copy()
print(copy_country_detail)
# Output:
{'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
C) items():
Returns a list containing a tuple with each key-value pair
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
print(country_detail.items())
#Output:
[('USA', 'DC'), ('Russia', 'Moscow'), ('India', 'Delhi')]
D) keys():
Returns a list containing only keys in the dictionary.
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
print(country_detail.keys())
#Output:
['USA', 'Russia', 'India']
E) values():
Returns a list only containing the values in the dictionary.
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
print(country_detail.values())
#Output:
['DC', 'Moscow', 'Delhi']
F) pop():
Remove the element with the specified key.
# python Dictionary methods
country_detail = {'USA': 'DC', 'Russia': 'Moscow', 'India': 'Delhi'}
1) print(country_detail.pop('Russia'))
#Output:
Moscow
2) print(country_detail)
#Output:
{'USA': 'DC', 'India': 'Delhi'}
In example 1, where we have used the pop function, first of all, the pop function requires an argument that is the key of the dictionary. This pop function returns the value of that key and simultaneously removes the key-value pair from the dictionary as you can see in the output of example 2.
Difference between a List and a Dictionary
List | Dictionary |
The list in Python is mutable. | Dictionary in Python is also mutable but keys don’t allow duplicate elements/ |
A list in Python is created by square brackets []. | A dictionary is created by using curly braces {}. |
Elements in the List can be reversed easily. | Elements in Python cannot be reversed because they are key-value pairs. |
Elements in the List are accessed by passing the index value. | Elements in the Dictionary are accessed by passing the key value. |
List in Python have the count() method to count the number of elements. | The dictionary does not contain count() method. |
FAQ
A dictionary in Python is an ordered collection (Python 3.7), before that, a dictionary in Python was an unordered collection. So, the dictionary is an ordered collection of items.
It stores the values in a key-value format, where the key is a unique identifier that is associated with each value.
Unlike other datatypes, a dictionary in Python can also hold a single value.
A dictionary can be created using two ways:
1) A dictionary can be created by using a sequence of elements inside the sequence of elements within the curly braces ({}) separated by a comma (,).
2) A dictionary in Python can be created by using the dict() function.
For a more understandable program, we should use dict() over {}, because it is easier to read.
1) Records of combined data can be found in a dictionary in Python.
2) Dictionary representations of sparse data structures are useful.
3) For Dictionary in Python, since these operations have already been done, there is no need to manually write data search algorithms.